I'll using llvm and c++ to make my own language on this Devlog
(want to use rust but I'm not used to rust yet)
And Big Thanks to Youtuber Toby Ho
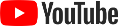
- What is LLVM?
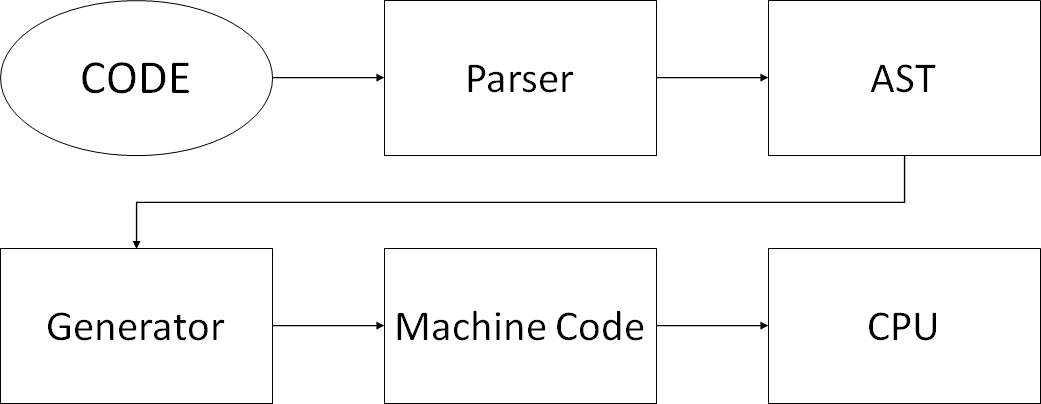
First of all the picture above is the way that normal programming language works
and today I'll try to follow the tutorial of the LLVM website
you can see the tutorials from
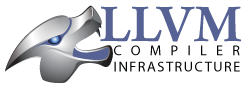
Now, How LLVM does work?
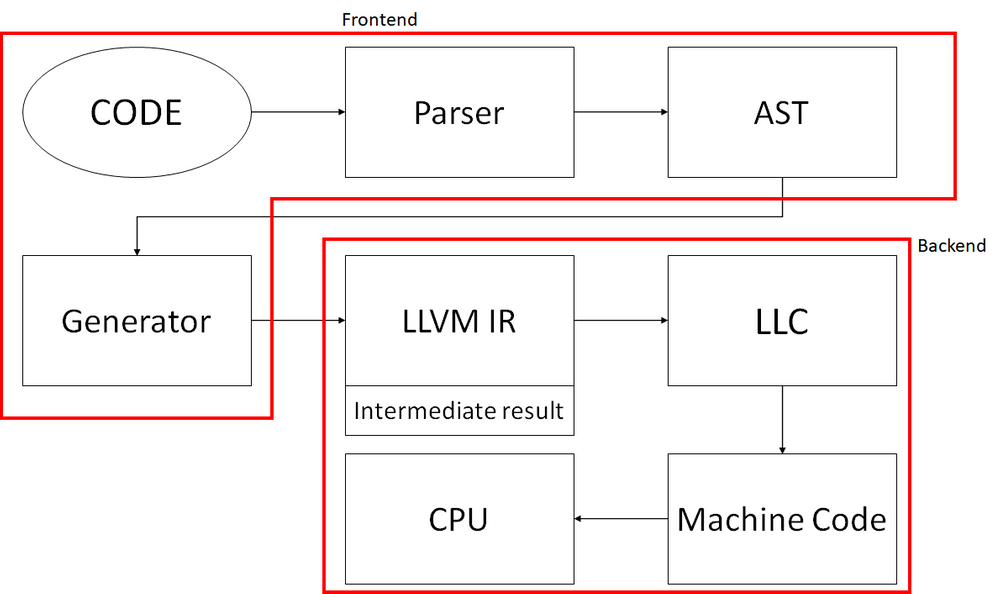
This is the simple ( I think so?) diagram that explaining the way how LLVM Works
and the fronted part is the area that the tutorial from the LLVM web (as I know you don't need to do the backend works)
- The Lexer
First, What is a Lexer?
Lexer is a kind of a function that is a parser that separate string of inputs into chunks
We will create the lexer first so, make a directory for the project
I'll call my Language as Nukleus-lang
mkdir Nukleus-lang
cat > nukleus.cpp
I have called my main lexer file as nukleus, just call it as you wish and press ctrl + d (Linux)
on mac or windows you can just do normal way of creating files like echo or just using the IDE that you using
After that open your IDE or editor (like VS code, Atom)
and follow the tutorial
#include <string>
enum Token{
tok_eof = -1,
tok_def = -2,
tok_extern = -3,
tok_identifier = -4,
tok_number = -5,
};
static std::string IdentifierStr;
static double NumVal;
static int gettok(){
static int LastChar = ' ';
//skip spaces
while(isspace(LastChar)){
LastChar = getchar();
}
//get identifier
if(isalpha(LastChar)){
IdentifierStr = LastChar;
while(isalnum((LastChar = getchar()))){
IdentifierStr += LastChar;
}
if(IdentifierStr == "def"){
return tok_def;
}
if(IdentifierStr == "extern"){
return tok_extern;
}
return tok_identifier;
}
if(isdigit(LastChar) || LastChar == '.'){
std::string NumStr;
do{
NumStr += LastChar;
LastChar = getchar();
}while(isdigit(LastChar) || LastChar == '.');
NumVal = strtod(NumStr.c_str(), 0);
return tok_number;
}
if(LastChar =='#'){
do{
LastChar = getchar();
}while(LastChar != EOF && LastChar !='\n' && LastChar != '\r');
if(LastChar != EOF){
return gettok();
}
}
if(LastChar == EOF){
return tok_eof;
}
int ThisChar = LastChar;
LastChar = getchar();
return ThisChar;
}
This is the thing that i typed
and for review that have I typed right?
for confirming process
int main(){
while(1){
int tok = gettok();
std::cout <<"got token: "<< tok << std::endl;
}
}
I have added these line to the bottom of the code and I have used g++ from my Ubuntu computer
g++ nukleus.cpp -o nukleus.bin
after that type
ls
Than you will see the two files, your-project-name.cpp, your-project-name.bin
Now let's test the lexer
./your-project-name.bin
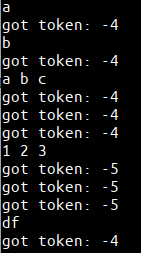